The HTML Document Object Model (DOM) defines a standard for accessing and manipulating HTML documents. The DOM is a World Wide Web Consortium (W3C) standard.
According to the W3C, the “W3C Document Object Model is a platform and language-neutral interface that allows programs and scripts to dynamically access and update the content, structure, and style of a document.”
The HTML DOM defines the objects and properties of all HTML elements, and the methods to access them. It provides a programmatic way to access, change, add, or delete HTML elements.
DOM Node Tree
The HTML DOM represents an HTML document as a tree-structure. The tree structure is also known as a node tree. All HTML nodes can be accessed through the node tree. Their contents can be modified or deleted, and new elements can be created. The node tree below shows the set of nodes and the connections between them.
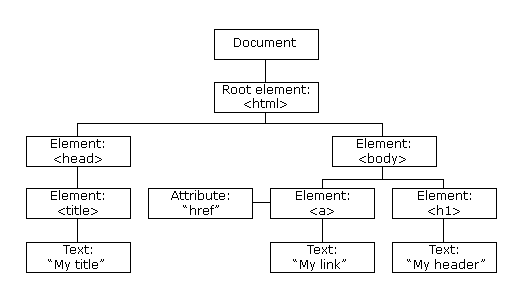
The nodes in the node tree have a relationship to each other. Parent nodes have children. Children on the same level are known as siblings. In an HTML document, the parent node is the <html>
element. It is also the root node.
Every node will have one parent except the root node. Any node can have one or more children nodes. A node with no children is known as a leaf node.
Common DOM Properties
Here is a list of the more common DOM properties you will be interested in using. The n
below represents a node.
n.attributes
– attribute nodes of noden.childNodes
– child nodes of noden.innerHTML
– text value of noden.nodeName
– name of noden.nodeValue
– value of noden.parentNode
– parent node of node
Common DOM Methods
There are also some DOM methods that are very useful. Here is a list that you can use:
n.appendChild(node)
– insert a child node to noden.getElementById(id)
– get the element with a specified idn.getElementsByTagName(name)
– get all elements with a specified tag namen.removeChild(node)
– remove a child node from node
Example
Let us take a look at a common example where you may want to update the text node for an HTML element in your document. We can do this programmatically using JavaScript, without reloading the web page.
<script type="text/javascript">
function updateText(x) {
document.getElementById('p1').innerHTML = x
}
</script>
<p id="p1">
Click on the link below to update this element.
</p>
<br />
<a href="JavaScript:void(0); updateText('Thank you for clicking me!');">
Click Me!
</a>
In this example, we used the getElementById
method to access the paragraph element with an id of p1
. We then set the text using the innerHTML
property. We could have used a different approach producing the same results. For example…
<script type="text/javascript">
function updateText(x) {
document.getElementById('p1').innerHTML = x
}
</script>
// <<REPLACE WITH>>
<script type="text/javascript">
function updateText(x) {
document.getElementById("p1").childNodes[0].nodeValue = x
}
</script>
As you can see, understanding how to access the nodes in the node tree can be very useful when working with JavaScript.