Paths drawn on an HTML5 Canvas is simply a series of points with drawing instructions between those points. For example, there can be a series of points defined on the canvas with lines or arcs drawn between them. Paths can be used to draw various types of shapes such as lines, circles, rectangles, and other multi-sided polygons.
To create a path onto the HTML5 Canvas, we can connect multiple subpaths. The ending point of each new subpath becomes the new context point.
We can use many methods to achieve this such as the lineTo()
, arcTo()
, quadraticCurveTo()
, and bezierCurveTo()
methods. We can also use the beginPath()
method each time we want to start drawing a new path and closePath()
.
Quadratic Curves
To draw a quadratic curve onto the HTML5 Canvas, we can use the quadraticCurveTo()
method.
<script>
context.quadraticCurveTo(controlX, controlY, endX, endY);
</script>
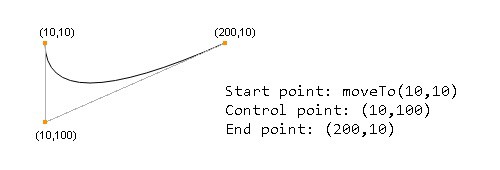
Quadratic curves are defined by the starting point, a control point, and an ending point.
<canvas id="myCanvas" width="500" height="250">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script>
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
context.beginPath();
context.moveTo(50, 50);
context.quadraticCurveTo(50, 150, 200, 50);
context.lineWidth = 3;
context.strokeStyle = '#FFAA00';
context.stroke();
</script>
bezierCurveTo()
To draw a bezier curve onto the HTML5 Canvas, we can use the bezierCurveTo()
method.
<script>
context.bezierCurveTo(controlX1, controlY1, controlX2, controlY2, endX, endY);
</script>
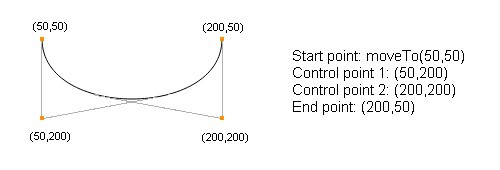
Bezier curves are defined by the starting point, two control points, and an ending point.
<canvas id="myCanvas" width="500" height="250">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script>
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
context.beginPath();
context.moveTo(50, 50);
context.bezierCurveTo(50, 200, 200, 200, 200, 50);
context.lineWidth = 3;
context.strokeStyle = '#FFAA00';
context.stroke();
</script>
Cloud Example
In this example, we will use bezier curves to draw a simple cloud.
<canvas id="myCanvas" width="500" height="250">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script>
var canvas = document.getElementById("myCanvas");
var context = canvas.getContext("2d");
var x = 125;
var y = 100;
context.beginPath();
context.moveTo(x, y);
context.bezierCurveTo(x - 40, y + 20, x - 40, y + 70, x + 60, y + 70);
context.bezierCurveTo(x + 80, y + 100, x + 150, y + 100, x + 170, y + 70);
context.bezierCurveTo(x + 250, y + 70, x + 250, y + 40, x + 220, y + 20);
context.bezierCurveTo(x + 260, y - 40, x + 200, y - 50, x + 170, y - 30);
context.bezierCurveTo(x + 150, y - 75, x + 80, y - 60, x + 80, y - 30);
context.bezierCurveTo(x + 30, y - 75, x - 20, y - 60, x, y);
context.closePath();
context.fillStyle = "#EFEFEF";
context.fill();
context.lineWidth = 3;
context.strokeStyle = '#AAAAAA';
context.stroke();
</script>