Configuring your div elements to the same height on your web page is a fairly simple task to accomplish with the help of a few lines of JavaScript code. Without help from JavaScript, the divs on the page will have different heights based on the content within them.
Setting the height manually is generally not a viable option because predicting the height needed for the content to fit in the div is not going to be known. This is especially true for blog sites where you may have a content and sidebar type of setup. The height of the content div will vary as new blog posts are published.
Webpage Example
The web page in this example has various components such as a header, content, sidebar, and footer. For the examples shown in this tutorial, the CSS code listed here is applied to the various HTML elements. As you can see, the main and sidebar divs are being placed side by side.
CSS Style
#wrapper {
width:500px;
margin:0 auto;
border:1px solid #ABABAB
}
#header {
background-color:#DADADA;
height:40px;
padding:20px;
font-size:36px;
border-bottom:1px solid #ABABAB;
}
#footer {
background-color:#DADADA;
height:25px;
padding:10px;
border-top:1px solid #ABABAB;
}
#main {
width:325px;
float:left;
background-color:#CCD9FF;
padding:15px;
}
#sidebar {
overflow:hidden;
background-color:#E5ECFF;
padding:15px;
}
#clear {
clear:both;
}
a {
display:block;
}
HTML Code
<!DOCTYPE html>
<html>
<head>
<title>Demo</title>
</head>
<body>
<div id="wrapper">
<div id="header">My Website</div>
<div id="main">
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore
et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut
aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum
dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui
officia deserunt mollit anim id est laborum.
</p>
<p>
Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam
rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt
explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia
consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt.
</p>
</div>
<div id="sidebar">
<a href="#">Link 1</a>
<a href="#">Link 2</a>
<a href="#">Link 3</a>
<a href="#">Link 4</a>
<a href="#">Link 5</a>
<a href="#">Link 6</a>
<a href="#">Link 7</a>
<a href="#">Link 8</a>
<a href="#">Link 9</a>
<a href="#">Link 10</a>
</div>
<div id="clear"></div>
<div id="footer">Footer</div>
</div>
</body>
</html>
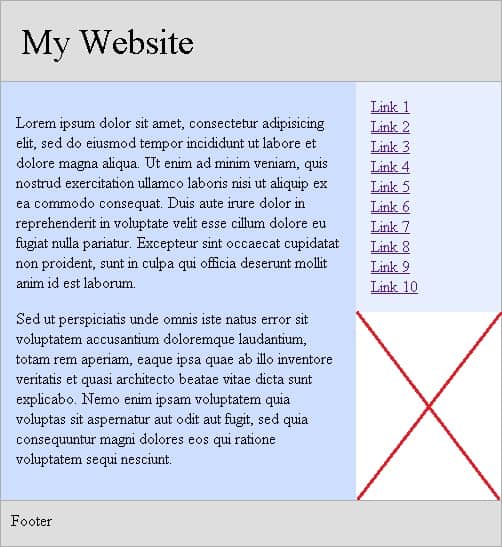
You will notice that there is an area under the sidebar that has no content. The area is represented by the red “x” in our figure. Since our div elements are not configured with a set height, the div elements will be sized according to the content they contain.
While we can manually set the height for the divs, this approach will only work well if the divs’ content is static in nature. For a website where the content is dynamic, we cannot set the height manually because we may not be aware of how much content will be placed within the div elements.
Resize Divs Dynamically
We will use a few lines of JavaScript code to resize the div elements so that they share the same height on the page. The results we want to achieve are shown in the following figure. In this case, the sidebar div is resized to match the height of the main div.
In the JavaScript code below, we will use a conditional statement to determine which div height is greater and resize the div with the smaller height to match the div with the greater height.
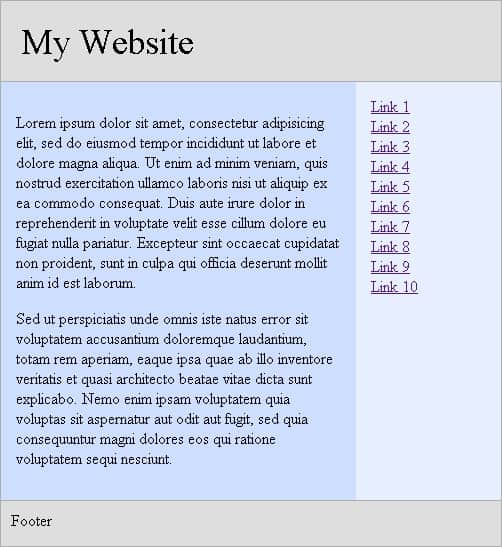
JavaScript Code
Place the following JavaScript code at the bottom of the web page, just before the closing </body>
tag.
<script>
function resizeDivs() {
var main = document.getElementById('main').offsetHeight;
var sidebar = document.getElementById('sidebar').offsetHeight;
if (sidebar > main) {
main = sidebar;
document.getElementById('main').style.height = document.getElementById('sidebar').style.height = main + 'px'
} else {
sidebar = main;
document.getElementById('sidebar').style.height = document.getElementById('main').style.height = sidebar + 'px'
}
}
window.onload = resizeDivs;
</script>